Except Valueerror Try Again in Python
Summary: in this tutorial, yous'll acquire how to use the Python try...except
statement to handle exceptions gracefully.
In Python, at that place're two main kinds of errors: syntax errors and exceptions.
Syntax errors
When yous write an invalid Python code, you'll get a syntax fault. For example:
electric current = 1 if current < x current += 1
Lawmaking linguistic communication: Python ( python )
If you try to run this code, you lot'll go the following mistake:
Lawmaking language: Crush Session ( shell )
File "d:/python/try-except.py", line 2 if current < ten ^ SyntaxError: invalid syntax
In this instance, the Python interpreter detected the error at the if
argument since a colon (:
) is missing after it.
The Python interpreter shows the file name and line number where the error occurred so that you can set it.
Exceptions
Fifty-fifty though when your code has valid syntax, information technology may cause an error during execution.
In Python, errors that occur during the execution are called exceptions. The causes of exceptions mainly come from the environment where the lawmaking executes. For example:
- Reading a file that doesn't exist.
- Connecting to a remote server that is offline.
- Bad user inputs.
When an exception occurs, the program doesn't handle it automatically. This results in an fault message.
For instance, the post-obit program calculates the sales growth:
# get input net sales impress('Enter the net sales for') previous = float(input('- Prior period:')) current = float(input('- Current menstruum:')) # calculate the alter in percent change = (current - previous) * 100 / previous # prove the result if change > 0: result = f'Sales increase {abs(modify)}%' else: result = f'Sales subtract {abs(change)}%' print(result)
Code language: Python ( python )
How it works.
- Commencement, prompt users for entering two numbers: the internet sales of the prior and electric current periods.
- Then, calculate the sales growth in percentage and show the outcome.
When you run the programme and enter 120'
as the internet sales of the current catamenia, the Python interpreter will issue the following output:
Lawmaking language: Beat Session ( trounce )
Enter the cyberspace sales for - Prior period:100 - Current period:120' Traceback (most recent telephone call concluding): File "d:/python/attempt-except.py", line 5, in <module> current = float(input('- Current menstruum:')) ValueError: could not convert cord to float: "120'"
The Python interpreter showed a traceback that includes detailed information of the exception:
- The path to the source code file (
d:/python/try-except.py
) that caused the exception. - The exact line of code that caused the exception (
line v
) - The statement that caused the exception
current = bladder(input('- Current period:'))
- The type of exception
ValueError
- The error message:
ValueError: could not convert string to float: "120'"
Considering bladder()
couldn't convert the string 120'
to a number, the Python interpreter issued a ValueError
exception.
In Python, exceptions accept unlike types such as TypeError
, NameError
, etc.
Treatment exceptions
To brand the program more than robust, you need to handle the exception once it occurs. In other words, y'all demand to catch the exception and inform users so that they can fix it.
A good way to handle this is non to prove what the Python interpreter returns. Instead, you replace that mistake message with a more user-friendly 1.
To do that, you lot tin can use the Python endeavour...except
statement:
try: # code that may cause error except: # handle errors
Lawmaking language: Python ( python )
The endeavour...except
statement works equally follows:
- The statements in the
try
clause executes get-go. - If no exception occurs, the
except
clause is skipped and the execution of theendeavour
statement is completed. - If an exception occurs at any statement in the
attempt
clause, the residue of the clause is skipped and theexcept
clause is executed.
The post-obit flowchart illustrates the endeavour...except
statement:
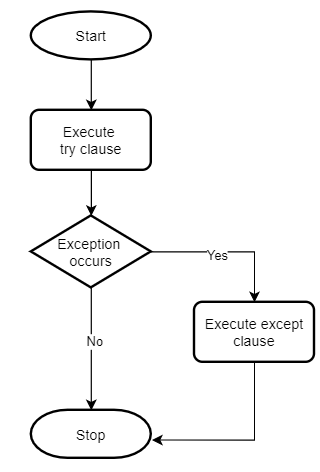
So to handle exceptions using the try...except
statement, you lot identify the code that may cause an exception in the try
clause and the code that handles exception in the except
clause.
Hither'due south how you can rewrite the program and uses the endeavour...except
statement to handle the exception:
try: # go input internet sales print('Enter the net sales for') previous = bladder(input('- Prior menses:')) current = bladder(input('- Current catamenia:')) # calculate the change in percentage change = (electric current - previous) * 100 / previous # show the result if modify > 0: result = f'Sales increase {abs(change)}%' else: event = f'Sales decrease {abs(change)}%' print(result) except: impress('Fault! Please enter a number for cyberspace sales.')
Lawmaking language: Python ( python )
If yous run the program again and enter the net sales which is not a number, the program will issue the bulletin that you specified in the except
cake instead:
Code linguistic communication: Shell Session ( beat )
Enter the net sales for - Prior menses:100 - Current menstruum:120' Error! Please enter a number for net sales.
Catching specific exceptions
When you enter the net sales of the prior catamenia as zippo, you'll get the following message:
Code linguistic communication: Shell Session ( shell )
Enter the internet sales for - Prior menses:0 - Current catamenia:100 Error! Please enter a number for net sales.
In this example, both net sales of the prior and current periods are numbers, the program yet issues an mistake message. Another exception must occur.
The endeavor...except
statement allows you to handle a particular exception. To catch a selected exception, y'all identify the type of exception subsequently the except
keyword:
endeavour: # code that may crusade an exception except ValueError equally error: # code to handle the exception
Lawmaking language: Python ( python )
For instance:
endeavour: # go input net sales print('Enter the net sales for') previous = float(input('- Prior menses:')) current = float(input('- Current catamenia:')) # summate the alter in percentage change = (electric current - previous) * 100 / previous # show the result if change > 0: result = f'Sales increase {abs(alter)}%' else: result = f'Sales decrease {abs(change)}%' print(result) except ValueError: impress('Error! Please enter a number for cyberspace sales.')
Code linguistic communication: Python ( python )
When you run a program and enter a string for the net sales, you'll become the same error bulletin.
However, if yous enter cypher for the net sales of the prior menstruation:
Code linguistic communication: Shell Session ( shell )
Enter the net sales for - Prior menstruum:0 - Current menstruum:100
… you'll go the post-obit mistake message:
Code linguistic communication: Trounce Session ( crush )
Traceback (most recent call last): File "d:/python/try-except.py", line ix, in <module> modify = (current - previous) * 100 / previous ZeroDivisionError: float division past zero
This time you got the ZeroDivisionError
exception. This division by goose egg exception is acquired by the post-obit statement:
alter = (current - previous) * 100 / previous
Code language: Python ( python )
And the reason is that the value of the previous
is zero.
Handling multiple exceptions
The try...except
allows you to handle multiple exceptions by specifying multiple except
clauses:
try: # lawmaking that may crusade an exception except Exception1 as e1: # handle exception except Exception2 equally e2: # handle exception except Exception3 as e3: # handle exception
Code linguistic communication: Python ( python )
This allows you to respond to each type of exception differently.
If you lot want to have the same response to some type of exceptions, you tin can group them in one except clause:
try: # code that may cause an exception except (Exception1, Exception2): # handle exception
Code language: Python ( python )
The following example shows how to employ the try...except
to handle the ValueError
and ZeroDivisionError
exceptions:
endeavor: # get input net sales print('Enter the net sales for') previous = float(input('- Prior menstruum:')) electric current = float(input('- Current menstruum:')) # calculate the change in per centum change = (current - previous) * 100 / previous # show the consequence if alter > 0: consequence = f'Sales increase {abs(change)}%' else: outcome = f'Sales decrease {abs(change)}%' print(result) except ValueError: print('Error! Please enter a number for net sales.') except ZeroDivisionError: print('Mistake! The prior internet sales cannot exist zero.')
Code linguistic communication: Python ( python )
When you enter zero for the internet sales of the prior period:
Lawmaking linguistic communication: Vanquish Session ( shell )
Enter the cyberspace sales for - Prior menstruation:0 - Current menstruation:120
… you lot'll get the post-obit error:
Code language: Crush Session ( beat out )
Error! The prior internet sales cannot be nothing.
It'due south a good exercise to take hold of other general errors by placing the catch Exception
block at the end of the list:
effort: # get input net sales impress('Enter the net sales for') previous = bladder(input('- Prior period:')) current = float(input('- Current menstruation:')) # calculate the change in percentage change = (current - previous) * 100 / previous # show the issue if alter > 0: event = f'Sales increase {abs(change)}%' else: result = f'Sales decrease {abs(modify)}%' impress(result) except ValueError: impress('Error! Please enter a number for net sales.') except ZeroDivisionError: print('Error! The prior net sales cannot be zero.') except Exception equally error: print(error)
Lawmaking language: Python ( python )
Summary
- Utilise Python
try...except
statement to handle exceptions gracefully. - Utilize specific exception in the
except
block equally much as possible. - Use the
except Exception
statement to catch other exceptions.
Did you observe this tutorial helpful ?
Source: https://www.pythontutorial.net/python-basics/python-try-except/
0 Response to "Except Valueerror Try Again in Python"
Post a Comment